Welcome to this tutorial on advanced techniques for socket programming in Go! If you’re already familiar with the basics of socket programming in Go, or if you’ve completed one of our previous tutorials on the subject, you’re ready to take your skills to the next level.
In this tutorial, we’ll explore some advanced concepts and techniques for building more powerful and scalable networked applications using Go sockets. We’ll start with a brief overview of the socket API in Go, and then dive into a few specific areas where you can use sockets to build more advanced systems.
The Go Socket API
The Go standard library provides a comprehensive set of APIs for working with sockets. The net package contains all of the functions and types you’ll need to create and use sockets, as well as a number of sub-packages for working with specific network protocols like TCP, UDP, and HTTP.
To get started with socket programming in Go, you’ll need to import the net package and use one of the Dial or Listen functions to create a socket. Here’s a simple example of how to create a client socket and connect to a server:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
| package main
import (
"fmt"
"net"
)
func main() {
// Dial a TCP socket to a server at a specific address
conn, err := net.Dial("tcp", "localhost:8080")
if err != nil {
// Handle error
}
// Send a message to the server
fmt.Fprintf(conn, "Hello, server!")
// Read a response from the server
buf := make([]byte, 1024)
n, err := conn.Read(buf)
if err != nil {
// Handle error
}
// Print the response
fmt.Println(string(buf[:n]))
// Close the connection
conn.Close()
}
|
To create a server socket, you can use the Listen function to bind to a specific address and port, and then use the Accept function to listen for incoming connections:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
| package main
import (
"fmt"
"net"
)
func main() {
// Bind a TCP socket to a specific address and port
ln, err := net.Listen("tcp", "localhost:8080")
if err != nil {
// Handle error
}
defer ln.Close()
for {
// Accept an incoming connection
conn, err := ln.Accept()
if err != nil {
// Handle error
}
// Read a message from the client
buf := make([]byte, 1024)
n, err := conn.Read(buf)
if err != nil {
// Handle error
}
// Print the message
fmt.Println(string(buf[:n]))
// Send a response to the client
fmt.Fprintf(conn, "Hello, client!")
// Close the connection
conn.Close()
}
}
|
Advanced Socket Techniques
Now that you have a basic understanding of how to create and use sockets in Go, let’s look at a few advanced techniques you can use to build more powerful and scalable networked applications.
Concurrent Server
One common pattern for building networked servers is to use concurrency to handle multiple clients simultaneously. In Go, you can use goroutines and channels to handle multiple connections concurrently without blocking the main thread.
Here’s an example of a concurrent server that uses a goroutine to handle each incoming connection:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
| package main
import (
"fmt"
"net"
)
func handleConnection(conn net.Conn) {
// Read a message from the client
buf := make([]byte, 1024)
n, err := conn.Read(buf)
if err != nil {
// Handle error
}
// Print the message
fmt.Println(string(buf[:n]))
// Send a response to the client
fmt.Fprintf(conn, "Hello, client!")
// Close the connection
conn.Close()
}
func main() {
// Bind a TCP socket to a specific address and port
ln, err := net.Listen("tcp", "localhost:8080")
if err != nil {
// Handle error
}
defer ln.Close()
for {
// Accept an incoming connection
conn, err := ln.Accept()
if err != nil {
// Handle error
}
// Handle the connection in a goroutine
go handleConnection(conn)
}
}
|
Using this pattern, you can build servers that can handle a large number of concurrent connections without running into performance issues.
Non-Blocking I/O
By default, the Read and Write functions in Go’s socket API are blocking, which means they will wait until they are able to read or write data before returning. This can be a problem if you’re building a high-performance server that needs to handle a large number of connections concurrently.
To overcome this issue, you can use non-blocking I/O to allow your server to handle multiple connections without waiting for blocking I/O operations to complete. To do this, you’ll need to use the SetReadDeadline and SetWriteDeadline functions to set a timeout for read and write operations, and then use the IsTimeout function to check for timeouts.
Here’s an example of a server that uses non-blocking I/O to handle multiple connections concurrently:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
| package main
import (
"fmt"
"net"
"time"
)
func handleConnection(conn net.Conn) {
for {
// Set a deadline for reading data
conn.SetReadDeadline(time.Now().Add(time.Second))
// Read data from the client
buf := make([]byte, 1024)
n, err := conn.Read(buf)
if err != nil {
if netErr, ok := err.(net.Error); ok && netErr.Timeout() {
// The read operation timed out, so we'll just continue
continue
} else {
// There was an error other than a timeout, so we'll break out of the loop
break
}
}
}
// Print the message
fmt.Println(string(buf[:n]))
// Set a deadline for writing data
conn.SetWriteDeadline(time.Now().Add(time.Second))
// Send a response to the client
fmt.Fprintf(conn, "Hello, client!")
}
// Close the connection
conn.Close()
func main() {
// Bind a TCP socket to a specific address and port
ln, err := net.Listen("tcp", "localhost:8080")
if err != nil {
// Handle error
}
defer ln.Close()
for {
// Accept an incoming connection
conn, err := ln.Accept()
if err != nil {
// Handle error
}
// Handle the connection in a goroutine
go handleConnection(conn)
}
}
|
Using non-blocking I/O can significantly improve the performance of your socket-based servers, especially when handling a large number of concurrent connections.
UDP Sockets
In addition to TCP sockets, Go’s net
package also provides support for working with UDP sockets. UDP is a connectionless protocol that allows you to send and receive datagrams without establishing a connection. This makes it well-suited for real-time applications where low latency is important, such as online gaming or voice over IP (VoIP).
To use UDP sockets in Go, you’ll need to use the DialUDP
and ListenUDP
functions to create client and server sockets, respectively. Here’s an example of a simple UDP client and server:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
|
// UDP client
package main
import (
"fmt"
"net"
)
func main() {
// Dial a UDP socket
conn, err := net.Dial("udp", "localhost:8080")
if err != nil {
// Handle error
}
defer conn.Close()
// Send a message to the server
fmt.Fprintf(conn, "Hello, server!")
}
// UDP server
package main
import (
"fmt"
"net"
)
func main() {
// Bind a UDP socket to a specific address and port
addr, err := net.ResolveUDPAddr("udp", "localhost:8080")
if err != nil {
// Handle error
}
conn, err := net.ListenUDP("udp", addr)
if err != nil {
// Handle error
}
defer conn.Close()
for {
// Read a message from the client
buf := make([]byte, 1024)
n, _, err := conn.ReadFromUDP(buf)
if err != nil {
// Handle error
}
// Print the message
fmt.Println(string(buf[:n]))
// Send a response to the client
conn.WriteToUDP([]byte("Hello, client!"), addr)
}
}
|
UDP sockets can be a powerful tool for building real-time networked applications, but it’s important to note that they do not provide the same level of reliability as TCP sockets. Because UDP is a connectionless protocol, there is no guarantee that a datagram will be delivered, and there is no mechanism for retransmitting lost datagrams.
If you need to ensure reliable delivery of data, you may want to consider using TCP sockets or a higher-level protocol like HTTP or WebSockets. However, if low latency is more important than reliability, UDP can be a good choice.
WebSockets
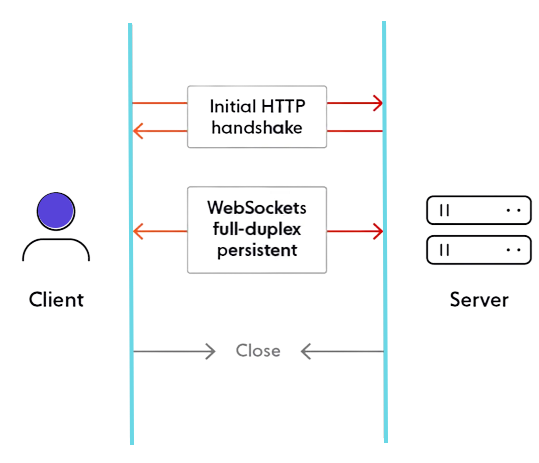
WebSockets are a web technology that provides a full-duplex communication channel over a single TCP connection. They are commonly used to build real-time web applications, such as chat systems or online games.
To use WebSockets in Go, you can use the websocket package in the golang.org/x/net repository. This package provides a comprehensive API for working with WebSockets, including support for handling the WebSocket handshake and framing messages.
Here’s an example of a simple WebSocket server that echoes back any messages it receives:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
| package main
import (
"fmt"
"log"
"net/http"
"golang.org/x/net/websocket"
)
func echoServer(ws *websocket.Conn) {
for {
// Read a message from the client
var msg string
err := websocket.Message.Receive(ws, &msg)
if err != nil {
// The connection was closed, so we'll break out of the loop
break
}
// Echo the message back to the client
err = websocket.Message.Send(ws, msg)
if err != nil {
// The connection was closed, so we'll break out of the loop
break
}
}
}
func main() {
http.Handle("/echo", websocket.Handler(echoServer))
log.Fatal(http.ListenAndServe(":8080", nil))
}
|
To connect to this server from a client, you can use the WebSocket constructor in JavaScript:
1
2
3
4
5
6
7
| const ws = new WebSocket('ws://localhost:8080/echo');
ws.onmessage = (event) => {
console.log(event.data);
};
ws.send('Hello, server!');
|
WebSockets can be a powerful tool for building real-time web applications, and the websocket package in Go makes it easy to work with them in your server-side code.
Conclusion
In this tutorial, we’ve explored some advanced concepts and techniques for socket programming in Go. We’ve looked at how to build concurrent servers, use non-blocking I/O, work with UDP sockets, and use WebSockets to build real-time web applications.
With these techniques in your toolkit, you’ll be well-equipped to build powerful and scalable networked applications using Go sockets.