Azure offers a variety of messaging options to help developers build reliable, scalable, and high-performance applications. These options include:
Azure Service Bus : A fully managed messaging service that provides reliable message delivery and support for a variety of messaging patterns, including point-to-point, publish-subscribe, and request-response.
Azure Event Grid : A fully managed event routing service that enables developers to build reactive and event-driven applications. Event Grid supports a wide range of event sources and can deliver events to various destinations, including Azure Functions, Azure Logic Apps, and Azure Event Hubs.
Azure Event Hubs : A scalable, real-time data ingestion and event processing platform that can handle millions of events per second. Event Hubs supports a variety of use cases, including real-time analytics, event-driven architectures, and IoT scenarios.
Azure Queue Storage : A scalable, reliable, and low-cost message queuing service that enables developers to decouple applications and build asynchronous workflows.
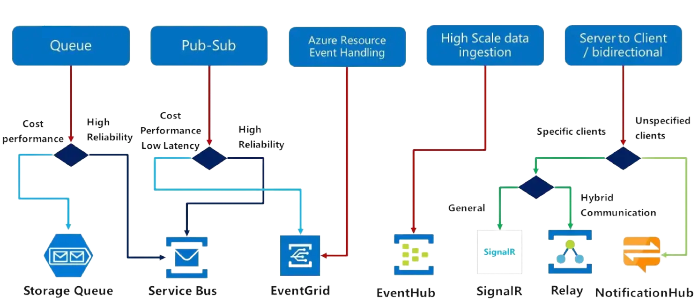
Below are some code samples that demonstrate how to use these messaging options in Azure:
Azure Service Bus
Azure Service Bus is a fully managed messaging service that provides reliable message delivery and support for a variety of messaging patterns, including point-to-point, publish-subscribe, and request-response.
Use cases:
- Asynchronous communication between applications
- Decoupling of services and microservices
- Real-time data processing and integration with other systems
To use Azure Service Bus, you’ll need to create a namespace and a queue or topic in the Azure portal. Then, you can use the following code to send and receive messages using the Azure Service Bus .NET SDK:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
| // Send a message to the queue
var connectionString = "Endpoint=sb://my-service-bus.servicebus.windows.net/;SharedAccessKeyName=RootManageSharedAccessKey;SharedAccessKey=xxxxxxx";
var queueName = "my-queue";
var client = new QueueClient(connectionString, queueName);
var message = new Message(Encoding.UTF8.GetBytes("Hello, world!"));
client.SendAsync(message).Wait();
// Receive a message from the queue
var message = client.ReceiveAsync().Result;
if (message != null)
{
var body = Encoding.UTF8.GetString(message.Body);
Console.WriteLine(body);
client.CompleteAsync(message.SystemProperties.LockToken).Wait();
}
|
Azure Event Grid
Azure Event Grid is a fully managed event routing service that enables developers to build reactive and event-driven applications. Event Grid supports a wide range of event sources and can deliver events to various destinations, including Azure Functions, Azure Logic Apps, and Azure Event Hubs.
Use cases:
- Event-driven architectures and serverless applications
- Automation of workflows and business processes
- Real-time analytics and data processing
To use Azure Event Grid, you’ll need to create an Event Grid topic in the Azure portal and then create a subscription to route events to a destination. Then, you can use the following code to publish events to the Event Grid topic using the Azure Event Grid .NET SDK:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
| // Publish an event to the Event Grid topic
var topicEndpoint = "https://my-event-grid-topic.westus2-1.eventgrid.azure.net/api/events";
var topicKey = "xxxxxxx";
var client = new EventGridClient(new TopicCredentials(topicKey));
var events = new List<EventGridEvent>
{
new EventGridEvent
{
Id = Guid.NewGuid().ToString(),
Subject = "my-event",
EventType = "my-event-type",
Data = new MyEventData { Message = "Hello, world!" },
EventTime = DateTime.UtcNow
}
};
client.PublishEventsAsync(topicEndpoint, events).Wait();
|
Azure Event Hubs
Azure Queue Storage is a scalable, reliable, and low-cost message queuing service that enables developers to decouple applications and build asynchronous workflows.
Use cases:
- Asynchronous communication between applications
- Decoupling of services and microservices
- Job scheduling and batch processing
To use Azure Event Hubs, you’ll need to create an Event Hubs namespace and an event hub in the Azure portal. Then, you can use the following code to send and receive events using the Azure Event Hubs .NET SDK:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
| // Send an event to the event hub
var connectionString = "Endpoint=sb://my-event-hubs-namespace.servicebus.windows.net/;SharedAccessKeyName=RootManageSharedAccessKey;SharedAccessKey=xxxxxxx";
var eventHubName = "my-event-hub";
var client = EventHubClient.CreateFromConnectionString(connectionString, eventHubName);
var eventData = new EventData(Encoding.UTF8.GetBytes("Hello, world!"));
client.SendAsync(eventData).Wait();
// Receive events from the event hub
var connectionString = "Endpoint=sb://my-event-hubs-namespace.servicebus.windows.net/;SharedAccessKeyName=RootManageSharedAccessKey;SharedAccessKey=xxxxxxx";
var eventHubName = "my-event-hub";
var client = EventHubClient.CreateFromConnectionString(connectionString, eventHubName);
var partitionIds = client.GetPartitionIdsAsync().Result;
foreach (var partitionId in partitionIds)
{
var receiver = client.CreateReceiver("$Default", partitionId, EventPosition.FromEnqueuedTime(DateTime.UtcNow));
var events = receiver.ReceiveAsync(100).Result;
foreach (var eventData in events)
{
var body = Encoding.UTF8.GetString(eventData.Body.Array);
Console.WriteLine(body);
}
}
|
Azure Queue Storage
Azure Queue Storage is a scalable, reliable, and low-cost message queuing service that enables developers to decouple applications and build asynchronous workflows.
Use cases:
- Asynchronous communication between applications
- Decoupling of services and microservices
- Job scheduling and batch processing
To use Azure Queue Storage, you’ll need to create a storage account and a queue in the Azure portal. Then, you can use the following code to send and receive messages using the Azure Storage .NET SDK:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
| // Send a message to the queue
var storageAccount = CloudStorageAccount.Parse("DefaultEndpointsProtocol=https;AccountName=mystorageaccount;AccountKey=xxxxxxx");
var queueClient = storageAccount.CreateCloudQueueClient();
var queue = queueClient.GetQueueReference("my-queue");
queue.AddMessageAsync(new CloudQueueMessage("Hello, world!")).Wait();
// Receive a message from the queue
var message = queue.GetMessageAsync().Result;
if (message != null)
{
var body = message.AsString;
Console.WriteLine(body);
queue.DeleteMessageAsync(message).Wait();
}
|
In conclusion, Azure offers a variety of messaging options to help developers build reliable and scalable applications. Whether you need a fully managed messaging service, an event routing service, a real-time data ingestion platform, or a simple message queuing service, Azure has you covered.